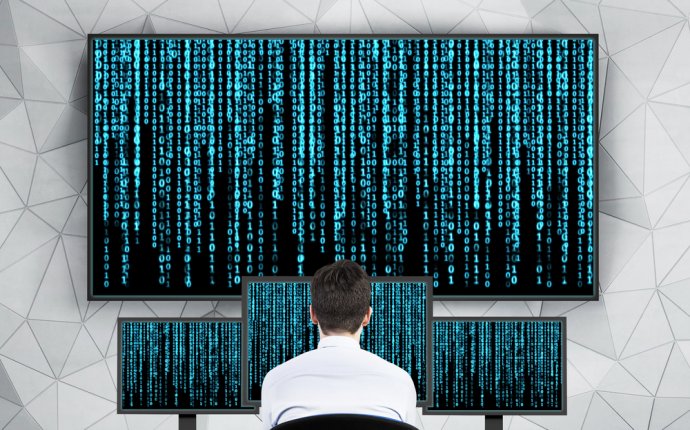
Bitcoin programming language
- Load the register with the value 23.
- Add 4b to the register, that is now 23 + 4b = 6e.
- Multiply the register by 1e, yielding 6e * 1e = ce4.
The register holds the final result, that is ce4.
Stack memory
Most of the time, we need to track complex program states with variables. In C, depending on whether a variable is allocated statically or with malloc, it’s stored in a differently arranged memory. While malloc-ed data is accessed like an element in a very big array, static variables are pushed to and popped from a pile of items called stack. A stack operates in a LIFO fashion (Last In First Out), meaning that the last item you push will be the first to pop out.
Consider this dummy function:
int foo { /* 1 */ /* 2 */ uint8_t a = 0x12; uint16_t b = 0xa4; uint32_t c = 0x2a5e7; /* 3 */ uint32_t d = a + b + c; return d; /* 4 */ }
The stack is initially empty (1):
Then, three variables are pushed (2):
[12] [12, a4 00] [12, a4 00, e7 a5 02 00]
A fourth variable is assigned the sum of the others and pushed onto the stack (3):
[12, a4 00, e7 a5 02 00, 9d a6 02 00]
The tip of the stack is the return value and is sent back to the function caller by other means. Each temporary stack variable is popped at the end of the block (4), because the push/pop operations must be balanced so that the stack always goes back to its initial state:
[12, a4 00, e7 a5 02 00] [12, a4 00] [12]
The Script machine code
Likewise, Bitcoin Core has its own “virtual processor” to interpret the Script machine code. Script features a rich set of opcodes, yet very limited compared to full-fledged CPUs like Intel’s, to name one. Some key facts about Script:
- Script does not loop.
- Script always terminates.
- Script memory access is stack-based.
In fact, point 1 implies 2. Point 3 means there’s no such thing like named variables in Script, you just do your calculations on a stack. Typically, the stack items you push become the operands of subsequent opcodes. At the end of the script, the top stack item is the return value.
Before presenting real world scripts, let’s first enumerate some opcodes. For a full set please check out the official